Well, coding officially starts on May 24, now is time supposed to read documentation, know your mentor(s) and discuss the project ideas, needs, requirements, difficulties, etc. but as many other GSoCers I couldn’t resist to code something.
My goal for the last week was to write a very simple Qt application showing the power of Grantlee. The idea was to simulate that I am reading a message in Kmail and I want to change the theme again and again.
You can take a look at the code in the soc-pim branch or:
svn co svn://anonsvn.kde.org/home/kde/branches/work/soc-pim/kdepim/examples/mail_grantlee/
Of course, you need to install Grantlee, before compiling the example:
git clone git://gitorious.org/grantlee/grantlee.git
cd grantlee
git checkout -b 0.1 origin/0.1
mkdir build && cd build
cmake ..
make && make install
Here some Kmail themes. I’m not an artist, so don’t expect too much, but I know some CSS and started creating the themes just for the example.
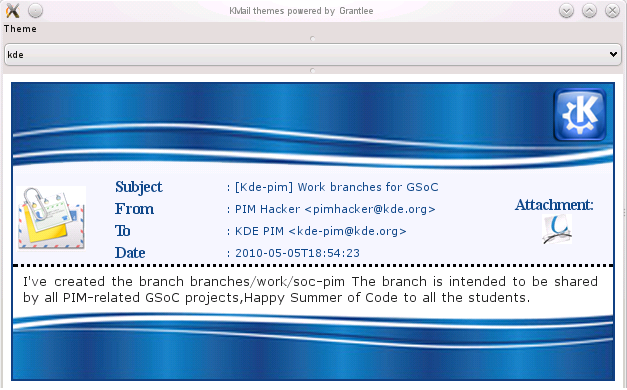


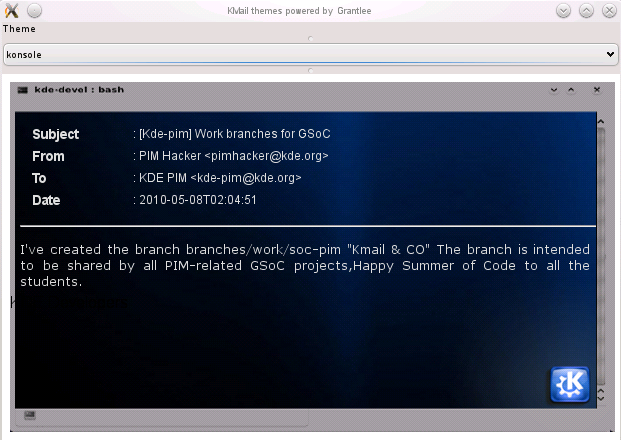
Don’t worry. Surely in the future there will be themes for everyone.
BTW, before continue, what is Grantlee?.
I talked about it in my GSoC proposal and Stephen has written a lot in his blog, but in case you haven’t read, here we go:
Grantlee is a Qt string template engine based on the Django template system. Django is a powerful Python framework that makes it easier to create web apps.
In general, what Grantlee does is that it allows an application separate logic from presentation. There are many benefits of doing this: Flexibility, clean code style (readability), consistency and beauty.
Developers concentrate on the technical aspects of the application (logic, performance,…) and artists work on the templates (HTML, CSS) and they both don’t need to touch each other’s code.
This separation allows the application to be built and tested independent of the visual presentation.
So, What would be the role of Grantlee in KDEPIM?
Let us see what is the job for the moment.
The MessageViewer is the library responsible for the header styling in some KDE PIM applications. If you browse the headerStyle class code, will see that there is HTML stuff everywhere. That makes hard, for both developer and artist, to change the way information is displayed.
That problem should be solved by integrating Grantlee with the MessageViewer and my task for now is to extract the presentation stuff, set up the Grantlee code and load the templates.
Of course the job is not only to load templates, I have in my mind all what can we achieve with this integration and believe me there is a lot to do. Grantlee has many cool features for theming, the API documentation is good and I will continue studying the capabilities during this community bounding period.
Well, it is very difficult to blog about all the Grantlee features in a post, but in case you are interested, you can have a quick overview of it here:
Grantlee for application developers.
Grantlee for theme artists.
Of course, I will be posting my grantlee adventures with KDEPIM week by week.
For example, this week I found a useful feature:
The ‘safe filter’. Imagine a mail message come with HTML content or special characters. We should render that message correctly in the template otherwise the content will look horrible.
The safe filter autoescapes a variable in case it has already been escaped. Just do:
{{ message|safe }}
That means, if a message comes with "Kmail & CO" ,that content will be rendered :
“Kmail & Co”
Filters affect the way variables are shown in a template. Grantlee comes with some default filters similar to Django like:
{{ variable|upper }} or {{ variable|cut:”something ” }} to cut a string from the variable. There are more filters and you can also create your own ones.
For the developer side, the fun thing is that you don’t need to rebuild your application if there is a change in the template.
For artists, the template syntax is really clear, very human readable.
I already started hacking a little on the headerStyle, but still I have nothing concrete so far to show you in a real world application. This is just the beginning. Althought, the MessageViewer code is huge, Thomas has given me good entry points to understand how it works.
The end result will be a MessageViewer without any presentation code and connected to a theme location.
The current default header styles are these:
Brief, Plain, Fancy, Enterprise and Mobile.
Please, if you are a KDE PIM user and want a change or feature on those styles, just tell me what to take into consideration before creating them. One feature we will provide is a user option to decide which header fields are displayed.
The second part of the GSoC project consists of GHNS integration in PIM applications that will use Grantlee, that way users can create and share their art-work. In the next weeks, we will be defining a standard package structure for those themes.
I consider theming an important part of the PIM module. On one side, users usually like to have their collection of beautiful themes, and on the other hand, it is also important for companies or educational institutions to show/export/print their identity (colors, logos, slogans).
In general, I’m happy understanding better and better how the project has to be carried on and my continuous Qt/KDE learning. If you have some feedback to provide, it will be well received.
I find the KDE community very friendly, that makes development, communication and the GSoC experience more enjoyable.
Hopefully, my mentors have passed the first week evaluation. I am really glad they are doing a great job and I am willing to help them with everything I can during the project.
Just kidding, they completely rock, their support and answers have helped me lot.
News about this integration coming soon.